Introduction
Falcon AI-powered Image Processing services detect undesirable images in near real-time providing 24/7 protection against the risks of user-generated content on your brand channels.
The Falcon API is a collection of data interpretation and analysis tools available as a web service that allows you to systematize the process of exploring and categorizing significant numbers of unstructured data.
Common use cases for using Falcon Image processing API include the following:
Version 1: In V1 you will be able to categorize your Images or videos into 2 different categories as Safe and Not Safe for work.
Version 2: In V2 you can get more detailed tags/labels for your image/video, including Explicit Nudity, Partial Nudity and Sexual Scene.
The Falcon API Flow
Using Falcon API is simple:
- 1. Click here if you haven't already and create an account.
- 2. Get API access credentials. To get your API credential, log in to your account and go to the API key page.
- 3. Submit your file to the API along with the necessary authentication details.
- 4. Receive an immediate response from the API that includes a comprehensive description of what was detected with the scores.
The API response provides all the necessary information in JSON format.
Image Processing
Falcon Image processing makes it simple to enhance image and video analysis to your application. You just provide an image or video to the Falcon Image Processing API, and the service can detect any improper content. Falcon Image Processing is based on the highly scalable, deep learning technology created by experts to analyze images and videos. It requires no machine learning knowledge to operate. Soon we will add other detection services to the API as well including facial analysis.
- Image formats: Jpeg, Jpg, Png, Gif, Webp & Bmp
- Maximum image size: 10MB
Content Moderation
Different communities require different rules, and the API makes it simple to establish the most effective ones. The API result enables you to create moderation rules that are tailored to your community and brand. For instance, partial nudity images might be permissible, while explicit nudity might not. Then you can filter out undesirable images based on your business policies using the predicted labels and their confidence scores returned by the API.
The format of the moderation outcome will be influenced by the API selected. For further information on the available APIs and the JSON response format, refer to the APIs Reference.
Head to the Quick start guide Falcon Image Moderation API:
Image Content Moderation, Version 1
Image Content Moderation, Version 2
Image Content Moderation, Version 2
The APIs accommodate the most common image formats including JPG, PNG, WebP, as well as animated multi-frame images like GIFs. The image must be transmitted as raw bytes, with a maximum size of 10MB for individual images or the entire bulk image group.
Version 1
- The Version 1 API is designed to simply categorize images in two categories as below:
- Not Safe for Work (NSFW)
- This category includes images and videos unsafe for work such as those related to porn, nudity, and genitals.
- Safe
- This category includes images that are completely safe without any sensitive nudity or pornographic scene.
Get Started
Create an account to obtain your own API keys if you haven't done so already.
Quick Setup
Getting started only requires a minimal amount of coding effort. Our goal has been to simplify and speed up the integration process for you.
Endpoint
https://api.nixofalcon.com/ai/image
Parameters
Parameter | Type | Description |
---|---|---|
Token | String | Client API Key |
Files | Object | Object Image to be sent for detection |
Code Example
- Python
- PHP
- cURL
headers = {'Accept': 'application/json', 'Authorization': 'Bearer API KEY' } files = {'image': (open('/path/to/image', 'rb'))} params = { 'model': 'content-moderation', 'version': '1' } response = requests.post('https://api.nixofalcon.com/ai/image', headers=headers, files=files, data=params) response.text
/** * requestAPI * Request service API * @param string $token API token generated in the project services * @param string $filePath full path to images * @param version $version service version, default to 1 * @param string $type Type of services default to 'im' service * @return bool */ function requestApi( string $token, string $filePath, int $version = 1, string $type = "im" ): bool { $url = "https://api.nixofalcon.com/ai/" . $type . "/v" . $version; $file = curl_file_create($filePath); $headers = ["Authorization: Bearer " . $token, "Accept: application/json"]; $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_HTTPHEADER, $headers); $data = [ "image" => $file, ]; curl_setopt($curl, CURLOPT_POSTFIELDS, $data); $response = curl_exec($curl); if (curl_errno($curl)) { echo "CURL ERROR - " . curl_error($curl); } curl_close($curl); return $response; } $apiKeys = "YOUR_API_KEY"; $filePath = "image/sample.jpg"; $version = 1; $serviceType = "im"; $result = requestApi($apiKeys, $filePath, $version, $serviceType);
curl -k -X POST "https://api.nixofalcon.com/ai/image" -H "Authorization: Bearer API Key" -H "Content-Type: multipart/form-data" -H "Accept: application/json" -F "image=@/path/to/image.jpg"
Response
The response JSON will contain a summary object, a confidence score for each label, and the moderation decision based on your configuration.
Version 2
This version of Falcon Image Moderation enables you to detect inappropriate content in milliseconds and allows you to filter the images based on your application requirements. The API provides a list of labels with confidence scores to enable fine-grained control over images you want to allow.
This API uses a two-level hierarchical taxonomy to label categories of inappropriate content. Each top-level category has several second-level categories that allow you more flexibility.
Labels available in Version 2 API:
- Sexual Scene
- Pornographic Scene
- Explicit Nudity
- Bare Chested Male
- Graphic Male Nudity
- Breast
- Graphic Female Nudity
- Partial Nudity
- Bikini or Lingerie
- Revealing Clothes
- Mini Skirt or Short
- Male Swimwear or Underwear
Get Started
Create an account to obtain your own API keys if you haven't done so already.
Quick Setup
Getting started only requires a minimal amount of coding effort. Our goal has been to simplify and speed up the integration process for you.
Endpoint
https://api.nixofalcon.com/ai/image
Parameters
Parameter | Type | Description |
---|---|---|
Token | String | Client API Key |
Files | Object | Object Image to be sent for detection |
Configuration
Image moderation Configuration simplifies the process of customizing rules and actions, accessible from your online dashboard. You have control over the analysis of each image and the criteria that determine acceptance or rejection.
To personalize the process flow, go to the Configuration section in your dashboard. There, you can choose the regulations that will be in place for each label and determine what steps should be taken as a result of those regulations - ACCEPT, REVIEW, or REJECT.
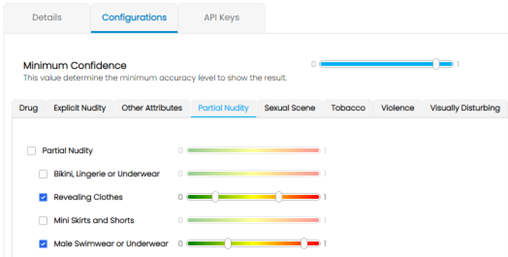
Note that once your configuration’s change has been submitted, it will apply immediately and reflect in the API response (Remark element).
Code Example
- Python
- PHP
- cURL
headers = {'Accept': 'application/json', 'Authorization': 'Bearer API KEY' } files = {'image': (open('/path/to/image', 'rb'))} params = { 'model': 'content-moderation', 'version': '2' } response = requests.post('https://api.nixofalcon.com/ai/image', headers=headers, files=files, data=params) response.text
/** * requestAPI * Request service API * @param string $token API token generated in the project services * @param string $filePath full path to images * @param version $version service version, default to 2 * @param string $type Type of services default to 'im' service * @return bool */ function requestApi( string $token, string $filePath, int $version = 2, string $type = "im" ): bool { $url = "https://api.nixofalcon.com/ai/" . $type . "/v" . $version; $file = curl_file_create($filePath); $headers = ["Authorization: Bearer " . $token, "Accept: application/json"]; $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_HTTPHEADER, $headers); $data = [ "image" => $file, ]; curl_setopt($curl, CURLOPT_POSTFIELDS, $data); $response = curl_exec($curl); if (curl_errno($curl)) { echo "CURL ERROR - " . curl_error($curl); } curl_close($curl); return $response; } $apiKeys = "YOUR_API_KEY"; $filePath = "image/sample.jpg"; $version = 2; $serviceType = "im"; $result = requestApi($apiKeys, $filePath, $version, $serviceType);
curl -k -X POST "https://api.nixofalcon.com/ai/image" -H "Authorization: Bearer API Key" -H "Content-Type: multipart/form-data" -H "Accept: application/json" -F "image=@/path/to/image.jpg"
Response
The response JSON will contain a summary object, a confidence score for each label, and the moderation decision based on your configuration.
Bulk Image Moderation
Even though, the easiest way to use the Image Moderation API is by submitting images individually, in some cases, it might be more efficient to submit multiple images at the same time.
Our API accepts that you submit multiple images at the same time within a single request.
Limitation
There is no limit on the number of images, however, the combined size of all images must not exceed 10 MB.
Code Example
Moderate three images through direct upload in one request.
- Python
- PHP
- cURL
headers = {'Accept': 'application/json', 'Authorization': 'Bearer API KEY'} files = { ('image[]': open('/path/to/image_01.jpg', 'rb')), ('image[]': open('/path/to/image_02.jpg', 'rb')), ('image[]': open('/path/to/image_03.jpg', 'rb')) } params = { 'model': 'content-moderation', 'version': '1' } response = requests.post('https://api.nixofalcon.com/ai/image', headers=headers, files=files, data=params) response.text
/** * requestAPI * Request service API * @param string $token API token generated in the project services * @param string $filePath full path to images * @param version $version service version, default to 1 * @param string $type Type of services default to 'im' service * @return bool */ function requestApi( string $token, string $filePaths, int $version = 1, string $type = "im" ): bool { $url = "https://api.nixofalcon.com/ai/" . $type . "/v" . $version; $data = []; foreach ($filePaths as $key => $filePath) { $data["image[$key]"] = curl_file_create($filePath); } $headers = ["Authorization: Bearer " . $token, "Accept: application/json"]; $curl = curl_init(); curl_setopt($curl, CURLOPT_URL, $url); curl_setopt($curl, CURLOPT_HTTPHEADER, $headers); curl_setopt($curl, CURLOPT_POSTFIELDS, $data); $response = curl_exec($curl); if (curl_errno($curl)) { echo "CURL ERROR - " . curl_error($curl); } curl_close($curl); return $response; } $apiKeys = "YOUR_API_KEY"; $filePaths = ["path/to/image_01.jpg", "path/to/image_02.jpg", "path/to/image_03.jpg"]; $version = 1; $serviceType = "im"; $result = requestApi($apiKeys, $filePaths, $version, $serviceType);
curl -k -X POST "https://api.nixofalcon.com/ai/image" -H "Authorization: Bearer API Key" -H "Content-Type: multipart/form-data" -H "Accept: application/json" -F "image[]=@/path/to/image_01.jpg" -F "image[]=@/path/to/image_02.jpg" -F "image[]=@/path/to/image_03.jpg"
Response
The API will provide a JSON response where the moderation outcomes for each image will be enclosed in separate fields. Using the "image" field within each object is crucial to link each image to its respective moderation result as the order cannot be guaranteed.
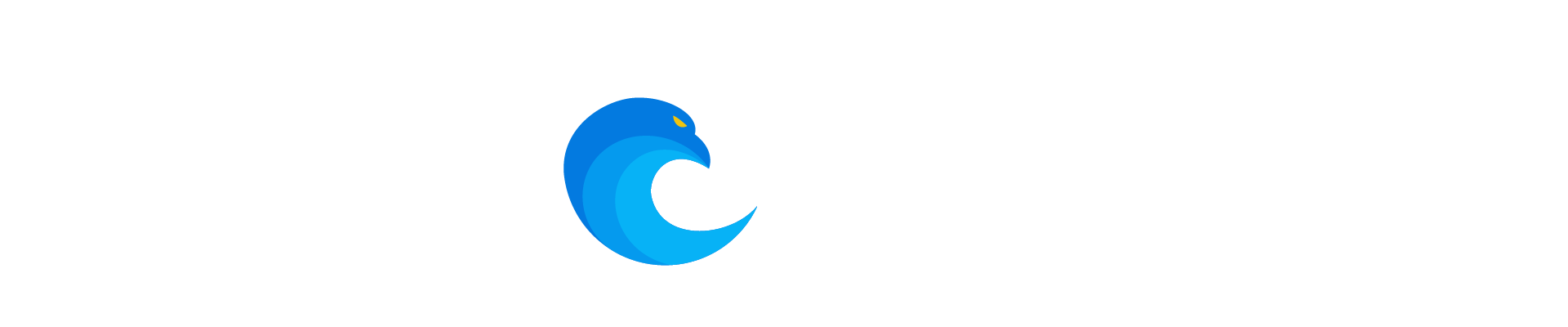
With Falcon robust models, you can identify inappropriate contents in images and videos in milliseconds, detect music in your audio files and filter those according to your business needs and requirements.
© 2025 Nixo Innovations Sdn Bhd. All rights reserved. Privacy Policy | Terms And Conditions
We use cookies to improve our product and our user’s experiences.
By continuing using our site you agree to our use of cookies.
Cookie Policies
Accept